In order to display Magento 2 Newsletter Popup manually – you will have to perform the following steps:
Select “Display Popup Method” = “Manually” in your Magento 2 backend as shown below:
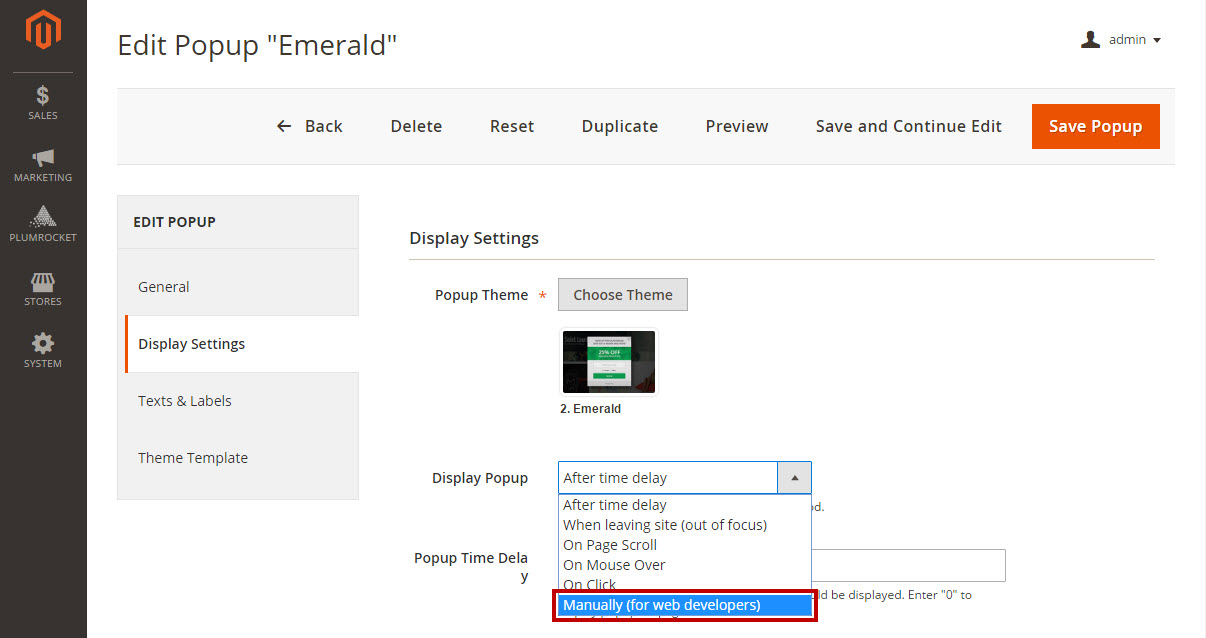
“Load” and “Show” newsletter popups using the following JavaScript methods below:
There are two available methods: .load() and .show(). “Load” method allows to load newsletter popup for Magento 2 content from server in background without displaying it. Then “Show” method will display the popup once it’s loaded.
.load()
Load popup without any arguments. This method is executed automatically when the extension is initialized.
.load([id][, callback ])
Load popup by specified newsletter popup ID. There is no need to check if the popup has been already loaded. This method will not load the same popup twice. You can find popup ID by going to your magento back-end, then Plumrocket -> Newsletter Popup -> Manage Newsletter Popups -> column “ID”.
If supplied, the callback is fired once the newsletter popup is loaded. The callback passed to .load() is guaranteed to be executed after the DOM is ready, so this is usually the best place to attach all other events and run other JavaScript code. It is hard to predict the overall load time of the newsletter popup from server and therefore we strongly recommend to use callback function. If the popup is already loaded on page then the callback will be executed immediately.
callback: function([bool status][, bool just_loaded])
Parameters
status — returns “true”, if the popup is available to be displayed, otherwise returns “false”.
just_loaded — returns “true”, if the popup was just loaded during this function call, otherwise returns “false” if it was previously loaded on page.
.show()
Display popup on the page after it was loaded with the .load() method. It’s an easy way to display popup without providing any arguments. If on the same page two popups are loaded – one using automatic “Display Popup Method” (such as “after the time delay” or “when leaving the site”) and the second one using the “manual” display method, then the automatically loaded popup will be displayed. If you have loaded two or more popups using the “manual” display popup method, then the first loaded popup will be displayed.
.show([id][, callback ])
Display popup by specified newsletter popup ID. This newsletter popup must be previously loaded using the .load() method. If a popup with a different “ID” is currently displayed on the page while the .show([id]) is being executed, then the previous popup will be hidden and the requested popup is displayed instead. There is no need to check for overlapping popups.
callback: function([bool status])
Parameters
status — returns “true”, if the popup exists and displayed on page, otherwise returns “false”.
EXAMPLES:
Example 1:
Let’s load & display newsletter popup with ID=5 and output a message to the Web Console using our callback function:
<script type="text/javascript">
prnewsletterPopup.load(5, function(status, just_loaded) {
if (just_loaded) {
console.log('Well done, Sir. The popup was just loaded.');
// alert('This is another successful newsletter load message!');
prnewsletterPopup.show(5);
}
}
</script>
Example 2:
Load and display any available newsletter popup. Please note that the “.load()” method without arguments is not required, it is executed automatically when the extension is initialized.
<script type="text/javascript">
prnewsletterPopup.show();
</script>
Example 3:
Load and display newsletter popup with ID=5 on link click
<script type="text/javascript">
prnewsletterPopup.load(5);
</script>
<a href="javascript:void(0);" onclick="return prnewsletterPopup.show(5)">
See Newsletter Popup
</a>
Example 4:
In this example, we are trying to display any default newsletter popup on page (no matter what ID). If this attempt was unsuccessful, then we will try to load and show popup ID=3.
<script type="text/javascript">
prnewsletterPopup.show(function(status) {
if (status == false) {
prnewsletterPopup.load(3, function(status) {
prnewsletterPopup.show();
});
}
});
</script>