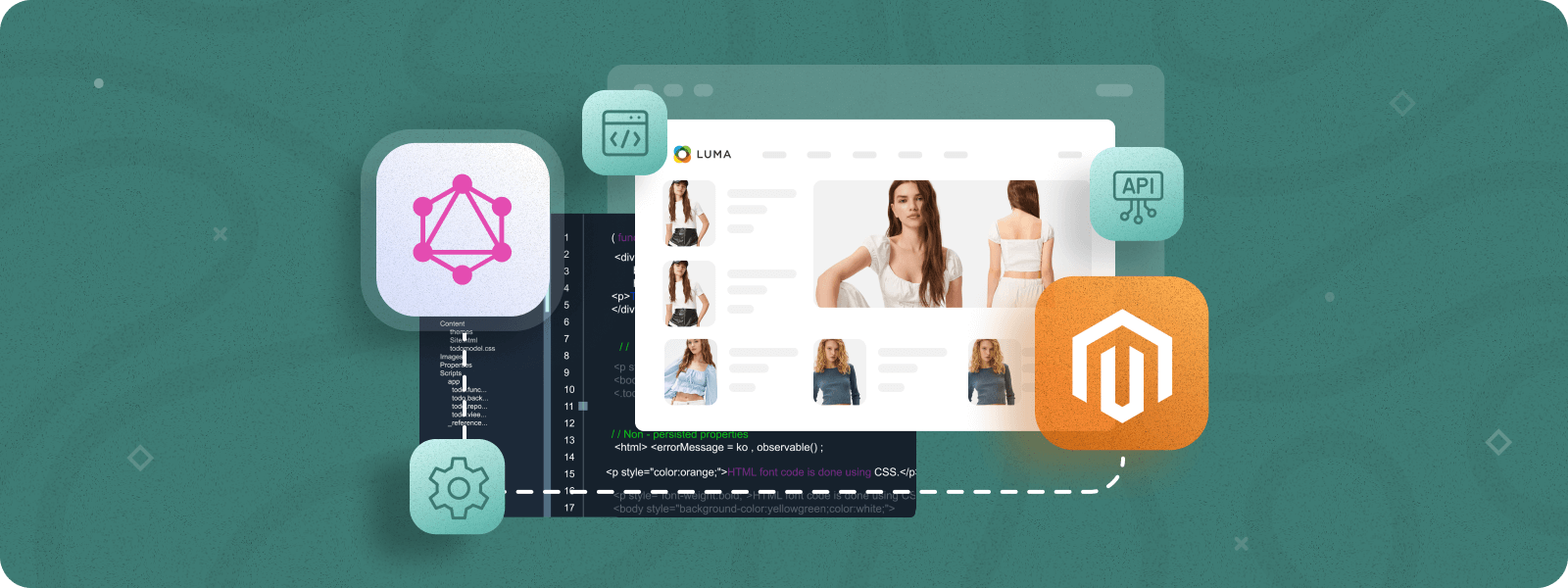
Ofen ecommerce websites deal with a wide range of data, which is essential for delivering products or services. To manage data efficiently and provide the best service, online stores need optimal solutions. Thus, ecommerce websites often use GraphQL to streamline their data fetching processes and ensure a seamless user experience.
In this tutorial, we’ll explore the fundamentals of GraphQL and compare it with REST. Also, look at the Magento 2 GraphQL query examples for the Size Chart Extension to gain insights into how GraphQL can be applied to various ecommerce scenarios.
What is GraphQL?
GraphQL is an API (Application Programming Interface) data query protocol. This language helps to query exactly the data you need and nothing more. GraphQL was developed by Facebook for their own APIs that they provide to their customers, but it quickly became used by other API developers.
What Differs GraphQL API from REST API
GraphQL and REST are often compared to each other because both are used to build and interact with API. However, GraphQL offers some advantages:
- Better performance: GraphQL is faster than REST because it doesn’t need multiple requests and minimizes data transfer by allowing clients to specify exactly what data they need.
- Fewer endpoints: in REST, each operation has its own endpoint, while in GraphQL there is only one.
- No data redundancy: in REST, the client receives all the data that is provided for a specific endpoint, even if there is no need for this. In GraphQL, the clients can specify what data they need, reducing the over-fetching and under-fetching of data.
GraphQL in Magento 2: How It Works
Schema definition
All operations in GraphQL are based on so-called schemas, which contain all available methods, entities, and fields that a user can retrieve. To retrieve the schema, you can use the following query:
query IntrospectionQuery {
__schema {
queryType {
name
}
mutationType {
name
}
subscriptionType {
name
}
types {
...FullType
}
}
}
fragment FullType on __Type {
kind
name
description
fields(includeDeprecated: true) {
name
description
args {
...InputValue
}
type {
...TypeRef
}
isDeprecated
deprecationReason
}
inputFields {
...InputValue
}
interfaces {
...TypeRef
}
enumValues(includeDeprecated: true) {
name
description
isDeprecated
deprecationReason
}
possibleTypes {
...TypeRef
}
}
fragment InputValue on __InputValue {
name
description
type {
...TypeRef
}
defaultValue
}
fragment TypeRef on __Type {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
ofType {
kind
name
}
}
}
}
}
}
}
}
Data query and mutations
Requests should be sent to the /graphql
endpoint ( {site-domain}/graphql
). This endpoint receives a list of data that the server is expected to send back to the client. These fields are structured hierarchically according to a defined schema.
In addition to querying data, Magento 2 GraphQL also supports mutations. These are special operations for creating, updating, or deleting data. For example, mutations are used to register users, change user data, add products to the cart, or create orders. Similar to queries, mutations allow a client to specify fields that should be returned after performing a mutation.
Execution Process
When the server receives a GraphQL request, it parses the query, validates whether the request matches the schema, and then passes it to the appropriate resolver to retrieve the data.
Data acquisition
In GraphQL, data is handled using resolvers. Each resolver function has its own responsibility for a specific field as defined in the schema. Resolvers can handle data from both the Magento 2 database and other external systems.
The resolver fetches the list of data that the client wants to receive, performs necessary data transformations, and returns the result to the GraphQL runtime, which then sends the response back to the client.
Respond
After receiving or processing data, the GraphQL server generates a response in JSON format and sends it back to the client. The structure of the response reflects exactly the structure of the request sent by the client.
Magento 2 GraphQL Query Examples for the Size Chart Extension
Query Example for Retrieving The Extension Status
The Size Chart extension allows you to check its configuration, specifically whether the module is enabled, using a standard Magento 2 GraphQL query. This query verifies if the extension is active in your store’s configuration. It is especially useful during setup, troubleshooting, or when integrating custom solutions that rely on the module’s functionality to ensure accurate operation.
type StoreConfig @doc(description: "The type contains information about a store config") {
pr_size_chart_enabled: Boolean @doc(description: "Define Plumrocket Size Chart module status")
}
query {
storeConfig {
pr_size_chart_enabled
}
}
{
"data": {
"storeConfig": {
"pr_size_chart_enabled": false
}
}
}
Query Example for Retrieving Product’s Size Chart
The extension provides a query to fetch detailed size chart information for a specific product. Use this query to fetch detailed size chart information for a specific product, including its content, styling, and display options. It is ideal for dynamically displaying tailored size charts on product pages, improving user experience, and ensuring accurate fit recommendations.
type Query {
prSizeChart (
productId: Int @doc(description: "Id of the Product") @doc(description: "The Size Chart query returns information about a Size Charts")
): SizeChart @doc(description: "The Size Chart query returns information about a Size Charts")
}
type SizeChart @doc(description: "Size Chart defines all Size Chart information") {
id: String @doc(description: "Identifier of the Size Chart")
name: String @doc(description: "Name of Size Chart")
content: String @doc(description: "Size Chart content")
display_type: String @doc(description: "Display type of Size Chart")
button_color: String @doc(description: "Size Chart button color")
button_border_color: String @doc(description: "Size Chart button border color")
button_text_color: String @doc(description: "Size Chart button text color")
button_icon: String @doc(description: "Size Chart button icon url")
}
query {
prSizeChart(productId: 1) {
id
name
content
display_type
button_color
button_border_color
button_text_color
button_icon
}
}
{
"data": {
"prSizeChart": {
"id": "1",
"name": "Men's Shoes",
"content": "<div class=\"window-title\"><h3>Men's Shoes</h3></div>\r\n<table class=\"table_size\">\r\n<tbody>\r\n<tr><th class=\"header-cell cell\">Men US</th><th class=\"header-cell cell\">EU</th><th class=\"header-cell cell\">UK</th><th class=\"header-cell cell\">CN</th></tr>\r\n<tr>\r\n<td class=\"cell\">5</td>\r\n<td class=\"cell\">38</td>\r\n<td class=\"cell\">4</td>\r\n<td class=\"cell\">38</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">5.5</td>\r\n<td class=\"cell\">38.5</td>\r\n<td class=\"cell\">4.5</td>\r\n<td class=\"cell\">39</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">6</td>\r\n<td class=\"cell\">39</td>\r\n<td class=\"cell\">5</td>\r\n<td class=\"cell\">39.5</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">6.5</td>\r\n<td class=\"cell\">39.5</td>\r\n<td class=\"cell\">5.5</td>\r\n<td class=\"cell\">40</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">7</td>\r\n<td class=\"cell\">40</td>\r\n<td class=\"cell\">6</td>\r\n<td class=\"cell\">41</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">7.5</td>\r\n<td class=\"cell\">40.5</td>\r\n<td class=\"cell\">6.5</td>\r\n<td class=\"cell\">41.5</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">8</td>\r\n<td class=\"cell\">41</td>\r\n<td class=\"cell\">7</td>\r\n<td class=\"cell\">42</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">8.5</td>\r\n<td class=\"cell\">41.5</td>\r\n<td class=\"cell\">7.5</td>\r\n<td class=\"cell\">43</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">9</td>\r\n<td class=\"cell\">42</td>\r\n<td class=\"cell\">8</td>\r\n<td class=\"cell\">43.5</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">9.5</td>\r\n<td class=\"cell\">42.5</td>\r\n<td class=\"cell\">8.5</td>\r\n<td class=\"cell\">44</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">10</td>\r\n<td class=\"cell\">43</td>\r\n<td class=\"cell\">9</td>\r\n<td class=\"cell\">44.5</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">10.5</td>\r\n<td class=\"cell\">43.5</td>\r\n<td class=\"cell\">9.5</td>\r\n<td class=\"cell\">45</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">11</td>\r\n<td class=\"cell\">44</td>\r\n<td class=\"cell\">10</td>\r\n<td class=\"cell\">45.5</td>\r\n</tr>\r\n<tr>\r\n<td class=\"cell\">12</td>\r\n<td class=\"cell\">44.5</td>\r\n<td class=\"cell\">10.5</td>\r\n<td class=\"cell\">46</td>\r\n</tr>\r\n<tr>\r\n<td colspan=\"4\">\r\n<p><strong><strong>Fiercely Fitted = Lorem Ipsum.<br /></strong></strong>Please check the measurement chart carefully.</p>\r\n</td>\r\n</tr>\r\n</tbody>\r\n</table>",
"display_type": "0",
"button_color": "#fffff",
"button_border_color": "",
"button_text_color": "#3399cc",
"button_icon": "https://app.mage246.dev/static/version1731363249/frontend/Magento/luma/en_US/Plumrocket_SizeChart/images/rule.png"
}
}
}
Conclusion
GraphQL in Magento 2 is a helpful tool for streamlining data fetching by enabling precise data queries and minimizing unnecessary data transfer. Compared to RESP APIs, GraphQL offers better performance, fewer endpoints, and reduced data redundancy, when compared to RESP API.
So, by incorporating GraphQL into your Magento 2 store, you can enhance performance, improve data fetching efficiency, and deliver a seamless shopping experience for your customers.