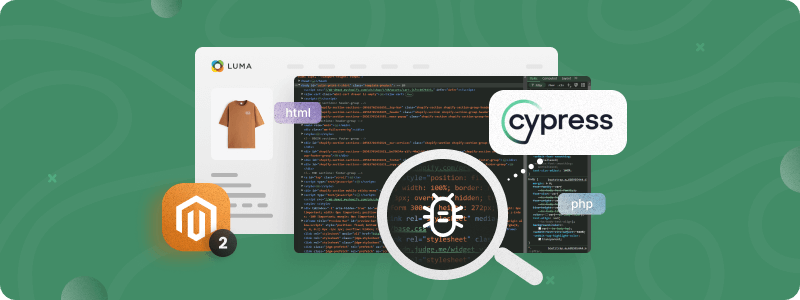
The testing process often takes some time and effort because of the increasing complexity of web applications. Fortunately, tools like Cypress can streamline this process by offering efficient E2E testing capabilities.
The E2E methodology allows for testing the overall customer journey from product browsing to checkout. This is especially effective for Magento 2 modules because the testing framework involves setting backend module configurations and checking the result on the frontend.
We prepared a hands-on example of creating and testing product labels in Magento 2. This provides you with an actionable example of using Cypress with Magento 2.
What is Cypress?
Cypress is a Javascript-based E2E testing framework that offers a solution for efficiently writing, running, and debugging automated tests. This framework is built for modern web applications and runs in the same browser to work directly with elements. Cypress can efficiently work with different tools and frameworks like React, Angular, Vue, and more.
Why Use Cypress for Magento 2 Testing?
Cypress is extremely helpful when working with Magento 2. Thanks to its features, you can conduct real-time testing to easily identify and debug issues. This process doesn’t take much time because Cypress uses automatic waiting mechanisms, streamlining Magento 2 test execution.
Moreover, Cypress uses E2E testing capabilities, which imitate user interaction with Magento 2 backend elements during their configuration. Specifically, they fill in the inputs, check the checkboxes, and click on the buttons when the elements are loaded and visible on the page for the user.
The same goes for the frontend: Cypress imitates user actions on this part, which helps test the Magento 2 frontend.
Features of Cypress
Cypress for Magento 2 has many benefits, such as easy setup, real-time reloads, and cross-browser testing, which can streamline the testing process and bring more results. In this tutorial, we prepared 3 core Cypress features that set this framework apart from others.
Automatic waiting
This Cypress feature constantly monitors the webpage for changes and waits until the necessary button becomes available. For example, some buttons may appear only after some element becomes visible or interactive, so to activate them, the testing framework should wait. Cypress regularly monitors the DOM for changes to ensure elements are prepared before test interactions, preventing test failures.
Interactive debugging
To inspect the test step-by-step, it is used interactive debugging. This functionality includes the ability to set breakpoints, examine variable values, and explore the application’s state at any given point. It helps effectively and quickly identify issues.
Time travel
One of the remarkable Cypress features is time travel, which helps to understand the sequence of events leading to a test failure. It steps through the test, examines variables, network requests, and DOM elements. Developers can step through history and pinpoint the exact moment when things went wrong.
Cypress Test Example: Creating and Verifying Product Labels in Magento 2
To test product labels on the frontend, you need to create a label with Cypress and verify its presence and content on the product grid.
let titleImage = 'image label'
let url = '' //You magento 2 domain
//Go to the Product Labels grid
cy.get('#add').click()
cy.get('.fieldset-wrapper-title').contains('General').should('be.visible')
cy.get('.page-title').contains('New Label Rule')
cy.get('#container', { timeout: 20000 }).should('be.visible')
cy.get('.admin__actions-switch [type="checkbox"]').check()
cy.get('.admin__field-control [name="title"]').type(titleImage)
cy.get('[name="priority"]').type('0')
cy.get('[name="store"]').select("All Store Views")
cy.get('[name="customer_group"]').select("ALL GROUPS")
cy.get('.admin__page-nav-link').contains('Display Settings').click()
cy.get('.fieldset-wrapper-title').contains('Display Settings').should('be.visible')
cy.get('.fieldset-wrapper-title').contains('Display Settings For Product List').should('be.visible')
cy.get('.admin__field .admin__field-control .admin__field.admin__field-option').contains('Image').click()
cy.get('[name="product_list[position]"]').select('Bottom Right')
cy.get('.accordion-btn').contains('Show more images').click()
cy.get('.gallery-wrapper .gallery-list').should('be.visible')
cy.get('[alt="c_0_25.svg"]').first().click()
cy.wait(500)
cy.get('.accordion-btn').contains('Show less images').click()
cy.get('.fieldset-wrapper-title').contains('Display Settings For Product Page').should('be.visible')
cy.get('.admin__page-nav-link').contains('Conditions').click()
cy.get('.legend').contains('Specify conditions when the product label').should('be.visible')
cy.get('.fieldset-wrapper-title').contains('Conditions').should('be.visible')
cy.get('.rule-param-children [title="Add"]').click()
cy.wait(500)
cy.get('[name="rule[conditions][1][new_child]"]').select('Category', {force: true})
cy.get('#conditions__1__children > li:nth-child(1) > span:nth-child(4) > a').click()
cy.get('[name="rule[conditions][1--1][value]"]').type('27, 4, 6')
cy.get('[alt="Apply"]').click()
cy.get('#save').click()
cy.get('.page-title').contains('Product Labels')
cy.get('.messages', { timeout: 20000 }).should('be.visible')
cy.get('.messages').contains('Label successfully saved!')
cy.get('td:nth-child(10)', { timeout: 20000 }).should('be.visible')
//Verify the created label on the Product Labels grid
cy.get('.admin__data-grid-wrap').contains(titleImage).parent().parent().as('manageLabels')
cy.get('@manageLabels').within(() => {
cy.get('td:nth-child(1)')
.find('input[type="checkbox"]')
.should('exist')
cy.get('td:nth-child(3)').contains(titleImage)
cy.get('td:nth-child(4) img[alt="' + titleImage + '"]').should('exist')
cy.get('td:nth-child(5) img[alt="' + titleImage + '"]').should('exist')
cy.get('td:nth-child(6)').contains('0')
cy.get('td:nth-child(7)').contains('Enabled')
cy.get('td:nth-child(10)').contains('Edit')
})
cy.get('.admin__data-grid-wrap').contains(titleImage).parent().parent().as('manageLabels')
cy.get('@manageLabels').within(() => {
cy.get('td:nth-child(1)')
.find('input[type="checkbox"]')
.should('exist')
cy.get('td:nth-child(3)').contains(titleImage)
cy.get('td:nth-child(4) img[alt="' + titleImage + '"]').should('exist')
cy.get('td:nth-child(5) img[alt="' + titleImage + '"]').should('exist')
cy.get('td:nth-child(6)').contains('0')
cy.get('td:nth-child(7)').contains('Enabled')
cy.get('td:nth-child(10)').contains('Edit')
This is a result of code. The label is created.
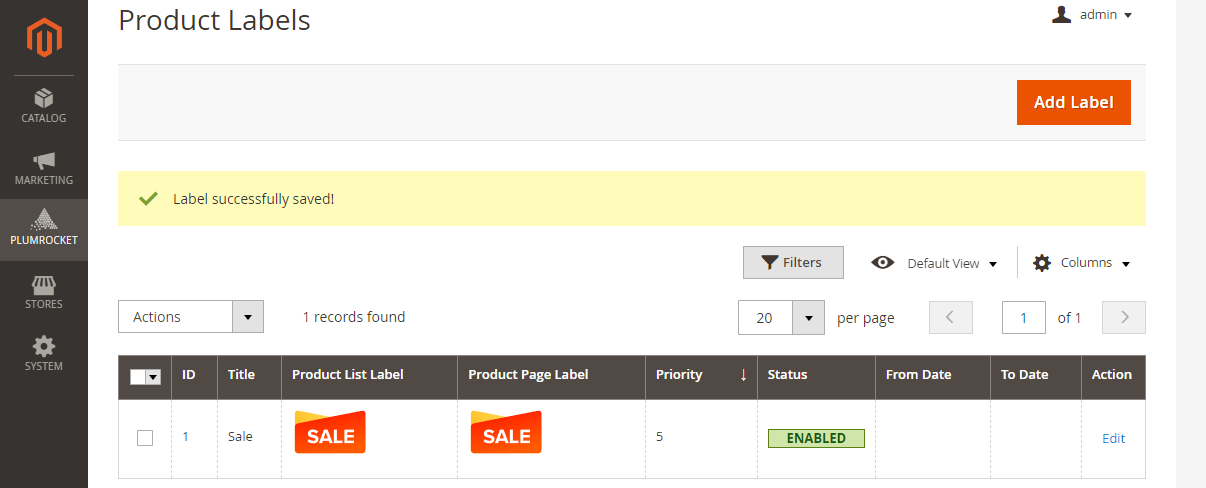
//Navigate to the frontend category page and verify if the specified label has appeared on the page
cy.visit(url + '/gear/watches.html')
cy.get('.item.product.product-item img[alt="' + titleImage + '"]').should('exist')
//From the category page, go to the product page and check whether the given label has appeared
cy.get('.item.product.product-item').first().click()
cy.get('.product.media img[alt="' + titleImage + '"]').should('exist')
//Go to a frontend page of another category and check whether the label we set is there
cy.visit(url + '/women/bottoms-women/pants-women.html')
cy.get('.item.product.product-item img[alt="' + titleImage + '"]').should('exist')
//From the category page, go to the page of another product and check whether the given label has appeared
cy.get('.item.product.product-item').first().click()
cy.get('.product.media img[alt="' + titleImage + '"]').should('exist')
Here is the result on the category page:
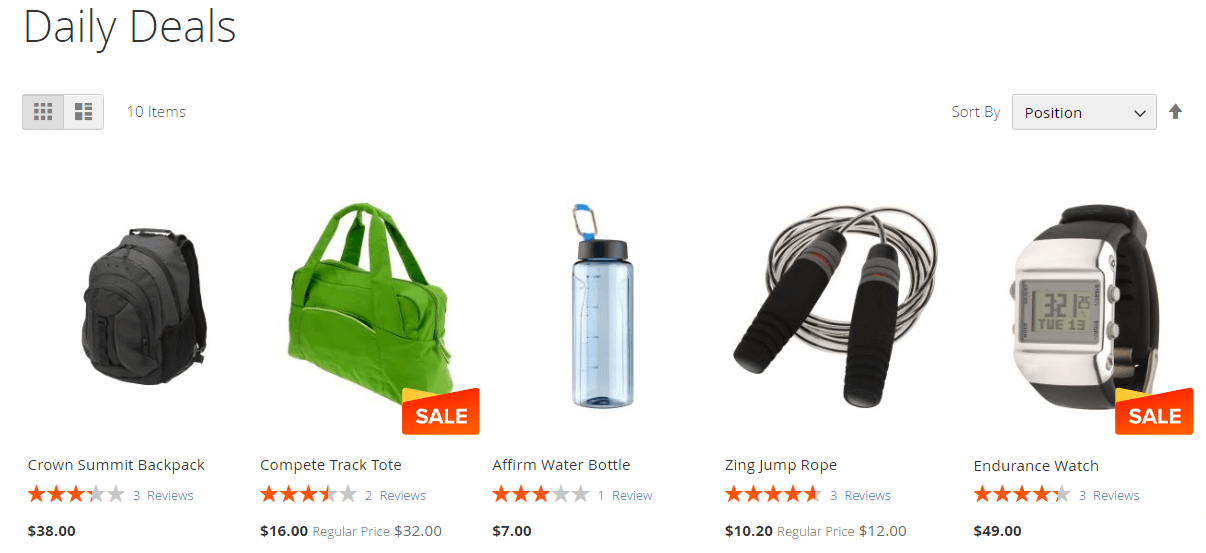
Here is the result on the product page:
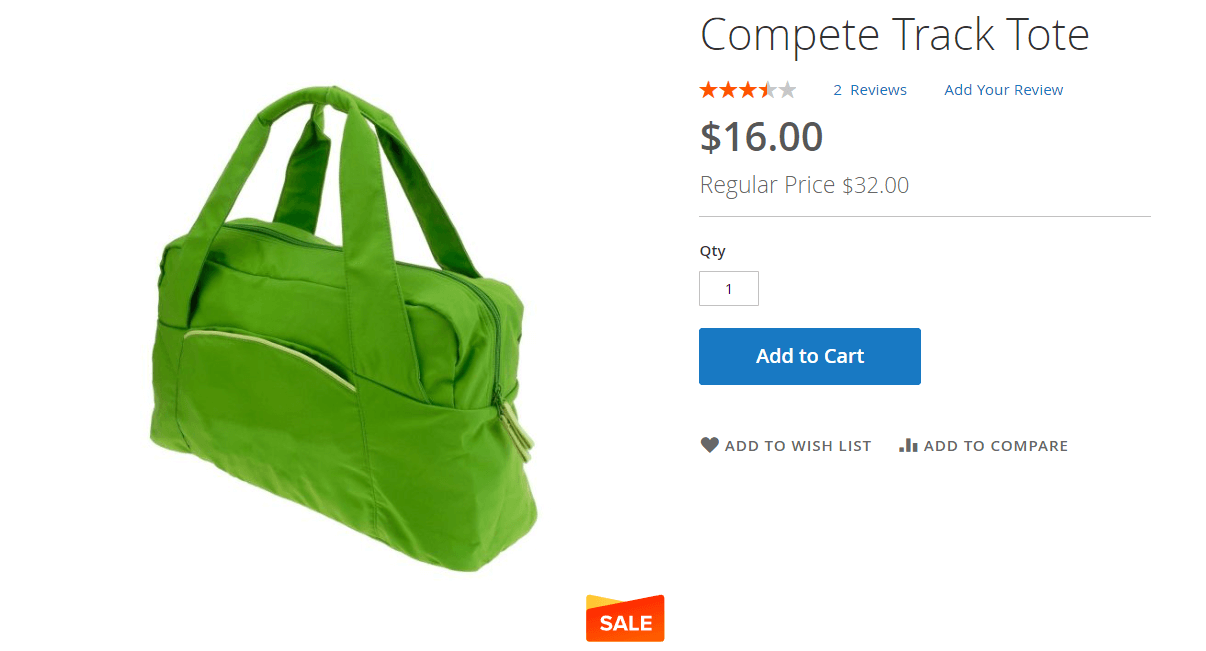
Cypress VS Alternative Tools for Magento 2 Testing
Although Cypress is considered to be the best tool for Magento 2 testing, it isn’t just one solution for enhancing the testing process. However, there are alternative tools, such as Playwright and Selenium WebDriver, which have their own benefits and advantages for QA engineers.
Playwright is a relatively new framework for web automation developed by Microsoft. While it supports multiple languages, it’s primarily focused on JavaScript and TypeScript. It also excels in cross-browser and cross-platform testing, providing reliable automation for Chrome, Firefox, WebKit, and mobile browsers. Playwright provides features such as code generation and automatic waiting, which makes it a helpful solution for testing.
Selenium WebDriver is an open-source tool for automating web application testing. This tool directly interacts with browsers like Firefox, Chrome, Safari, and Internet Explorer, so it is possible to execute cross-browser testing with Selenium. Also, WebDriver supports different languages such as Java, .Net, PHP, Python, Perl, and Ruby. However, compared to Playwright and Cypress, Selenium WebDriver is considered much slower.
While many tools have their own advantages, Cypress stands out with a user-friendly interface, detailed documentation, and easy-to-write tests. The most advantageous aspect is that this framework uses the E2E testing approach. By replicating the customer journey, it empowers testers to work more efficiently and effectively, accelerating development cycles.
Enhance your store’s performance with expert Magento development and high-quality QA services today!