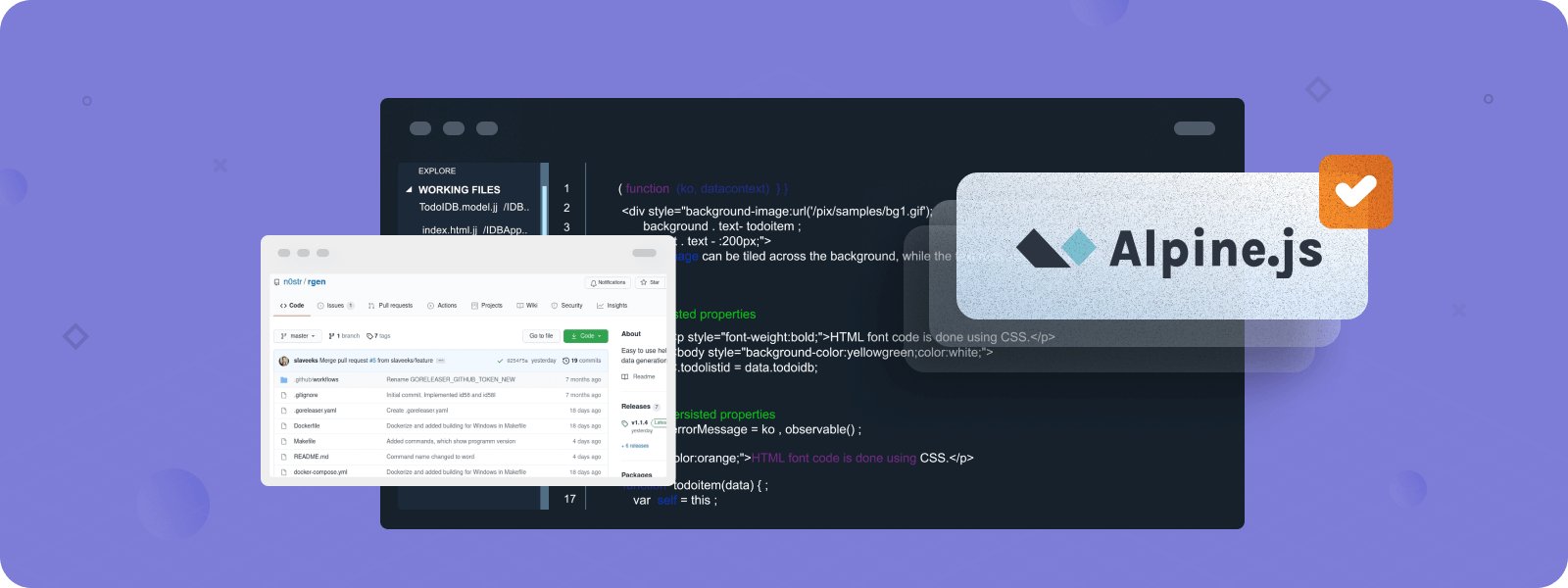
Alpine.js framework has ample opportunities for manipulating data transferred from the backend to the frontend. This tutorial dives into the details of using Alpine to work with arrays of data.
What is Alpine.js?
Alpine.js is a lightweight JavaScript library that adds interactivity to your web pages simply and hurdlessly. This makes Alpine.js an ideal choice for projects that need interactivity without complexity. Take Hyvä themes for example, a popular solution for building headless storefronts for Magento 2. Hyvä is built only on two lightweight technologies – Alpine.js and TailwindCSS – to create dynamic and interactive shopping experiences, all while preserving outstanding website speed.
Beyond simplicity, Alpine.js is a powerful tool for effectively working with data on the client side (user’s browser). This aspect simplifies the development process and improves user interaction with the website.
One of its remarkable features is the x-for
directive, which structures multidimensional arrays. This directive allows complex data to be easily displayed within web pages. This feature is necessary when rendering multidimensional arrays, so let’s discuss it in more detail.
What is ‘x-for’ Directive?
According to Alpine.js documentation, the x-for
directive allows the creation of DOM (Document Object Model) elements by iterating through an array. In other words, x-for
is an instruction that allows you to quickly loop through a huge list of items.
However, to have an error-free, clean, and efficient code, there are two requirements that you should follow when using this directive:
x-for
directive should be declared within the Alpine.jstemplate
tag. Thetemplate
tag helps define the structure for each repeated piece of data. This tag acts as a blueprint for generating HTML elements based on your data. Thus, the Alpine.jstemplate
tag is essential for separating dynamic content and static structure of your HTML and making it more organized.
- The
template
tag should contain only one child element. It is crucial to help Alpine.js identify exactly what element should be repeated for each item in your data. The single child element reduces confusion and avoids errors in the rendering process.
How to Work with One-Dimensional Arrays
A simple example of using the Alpine.js template element with the x-for
directive is rendering a one-dimensional array of data.
For example, let’s place a variable in the x-data directive that represents an array of user activity:
<div x-data="{ activities: [‘Create’, ‘Edit’, ‘Delete’] }">
<template x-for="activity in activities">
<div x-text=”activity”></div>
</template>
</div>
The result of this code snippet executing will be three separate blocks:+
<div> Create </div>
<div> Edit </div>
<div> Delete </div>
How to Use Alpine.js Nested Template Components for Rendering Two-Dimensional Array of Data
Rendering multidimensional data arrays with Alpine.js is only possible when using nested template components. To show how it works, let’s render two-dimensional data about user activities by day from the backend.
In this case, we need to render days (which are objects with properties) and user activities (which are property values and objects at the same time). Since this data is a two-dimensional array, we need to use Alpine.js nested components to successfully render it on the frontend.
<div x-data="{ activitiesByDays: … }">
<template x-for="(activities, day) in activitiesByDays">
<p x-text=”day”></p>
<template x-for="activity in activities">
<div x-text”activity.title”></div>
</template>
</template>
</div>
However, the code snippet above will not work as expected – only the names of days will be displayed. That’s because of the second x-for
directive requirement mentioned earlier – the Alpine.js template tag should contain only one child element.
The solution to this problem is to wrap all nested elements of a template tag in a <div> tag.
<div x-data="{ activitiesByDays: … }">
<template x-for="(activities, day) in activitiesByDays">
<div>
<p x-text=”day”></p>
<template x-for="activity in activities">
<div x-text”activity.title”></div>
</template>
</div>
</template>
</div>
Now, the information will be rendered as expected — after the day’s name, there will be a list of user activities.
Conclusion
Alpine.js is a very helpful tool for rendering data on the client side, making the page dynamically updated and interactive. In this tutorial, we explored in detail how the x-for
directive allows you to iterate over the elements of an array and render data without having to manually manipulate the HTML structure.
Additionally, we highlighted the key aspects when using the Alpine.js template to work with data:
- How to easily use
x-for
to output simple one-dimensional arrays of data. - How to render more complex structures, such as two-dimensional arrays, using nested template components.
- The most common issue with data rendering via the
x-for
directive.
If you have any difficulties, feel free to reach out to us for professional custom development services. We will be happy to help!