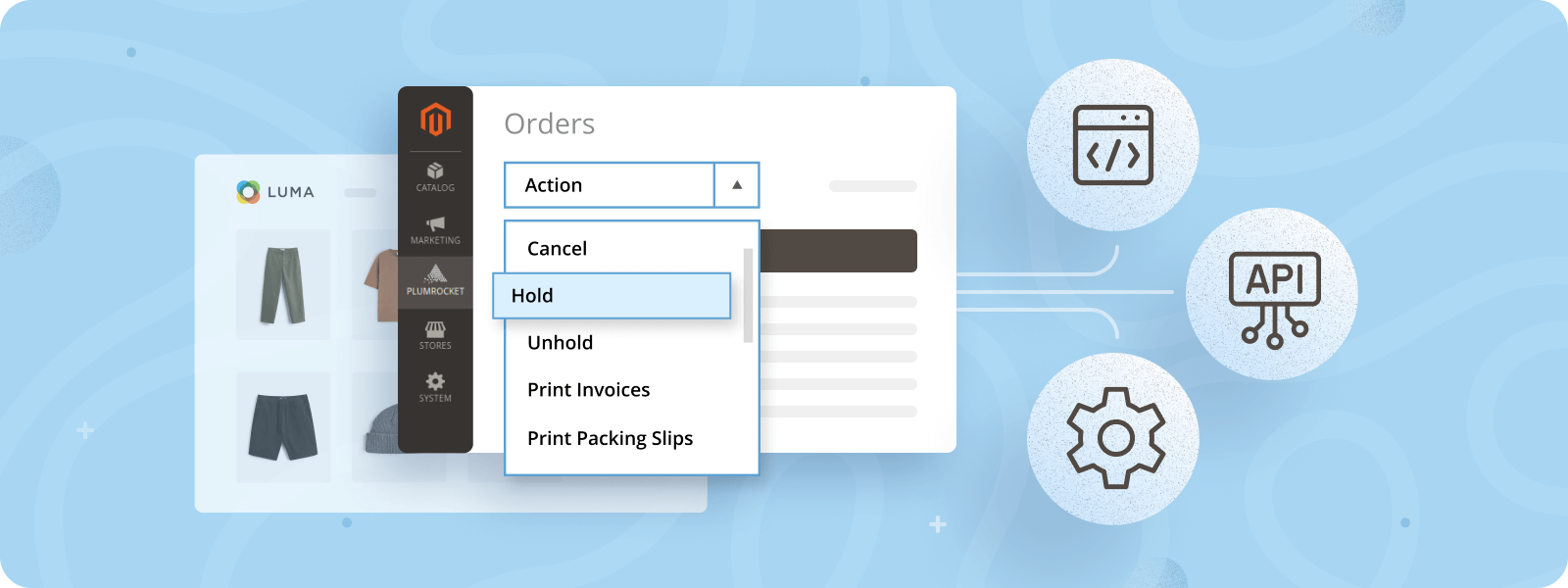
Magento 2 offers a comprehensive order management system with 14 predefined order statuses, including: Received, Suspected Fraud, Processing, Pending Payment, Payment Review, Pending, On Hold, Complete, Closed, Canceled, Rejected, PayPal Canceled Reversal, Pending PayPal, PayPal Reversed.
These statuses help merchants track the progress of each order through the fulfillment process.
There are several ways to change order status in Magento 2, depending on your requirements:
- Manually through the Magento Admin panel
- Programmatically using custom code
- Via Magento REST API
- Automatically using Magento 2 extensions
In this article, we will walk you through each of these methods. Choose the method that best aligns with your specific needs and technical capabilities.
How to Change Order Status in Magento 2 Manually in the Admin Panel
In the Magento 2 admin panel, you can only manually change the order status to On Hold or Canceled. All other statuses depend on payment method, payment status, shipping, and refunds.
Steps to change order status to Canceled or On hold status:
Step-by-step guide:
- Open the Magento 2 Orders grid page.
- Use checkboxes to select the orders you want to change the status of.
- Choose the Cancel or Hold option from the Actions drop-down.
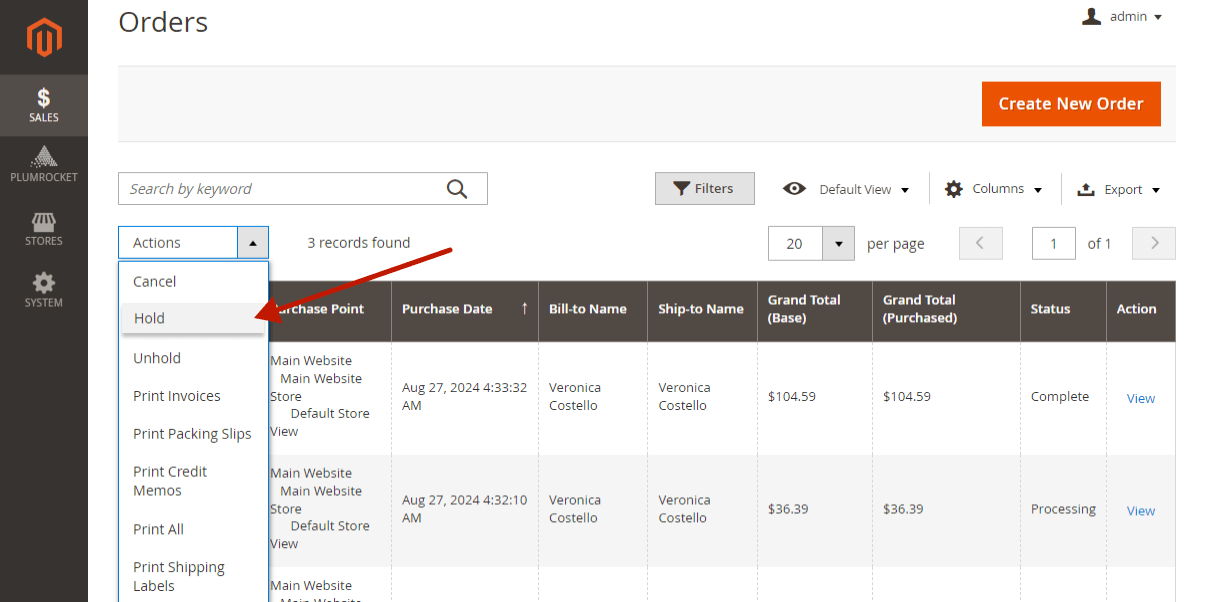
How to Change Order Status in Magento 2 Programmatically
We will create a Magento 2 model CompleteOrder
that will change the order status to Complete. It leverages the Magento\Sales\Model\Order
model to retrieve the order by its ID, update the order’s state and status, and then save the changes.
<?php
namespace Vendor\CompleteOrder\Model;
use Magento\Sales\Model\Order;
class CompleteOrder
{
private $order;
public function __construct(Order $order)
{
$this->order = $order;
}
public function execute($orderId)
{
$order = $this->order->load($orderId);
$order->setState(Order::STATE_COMPLETE)
->setStatus(Order::STATE_COMPLETE)
->save();
}
}
The code below shows how you can use the model. Simply provide the order ID as an argument to the execute method, and the class will handle the rest.
$orderComplete = $objectManager->create(\Vendor\CompleteOrder\Model\CompleteOrder::class)
$orderId = 1;
$orderComplete->execute($orderId);
This is a simple code example of how to change order status programmatically in Magento 2. However, in real-world scenarios, it’s important to consider the current status of the order before changing it to Complete. Depending on your business process, you may need to create a shipment or generate an invoice before setting the order as completed.
How to Change Order Status in Magento 2 (REST API Method)
To update the order status via Magento 2 Rest API, follow these steps:
- Get Bear access token from the Magento store using the following request:
curl -X POST "https://magento-store.com/rest/V1/integration/admin/token" \
-H "Content-Type: application/json" \
-d '{
"username": "admin_username",
"password": "admin_password"
- Replace the placeholders with the actual token and with the specific order ID in the request below:
curl -X POST "https://magento-store.com/rest/V1/orders" \
-H "Content-Type: application/json" \
-H "Authorization: Bearer <access-token>" \
-d '{
"entity": {
"entity_id": <orderId>,
"status": "canceled",
"state": "canceled"
}
}'
By using this Magento 2 API, you can also change other order fields, making it a powerful tool for order management.
How to Change Order Status in Magento 2 Automatically
One efficient way to automate order status updates in Magento 2 is by using the Magento 2 Auto Invoice & Shipment Extension. This extension can automatically generate invoices and shipments, which triggers an automatic change in the order status to Processing or Complete, depending on the fulfillment stage.
Key features of this extension include:
Bulk Invoice and Shipment
The extension enables store admins to generate Magento invoices and shipments in bulk, saving time and streamlining order processing.
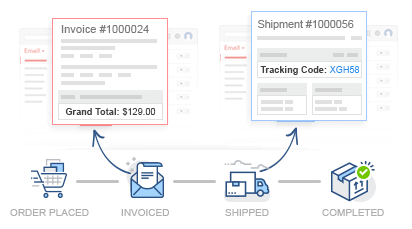
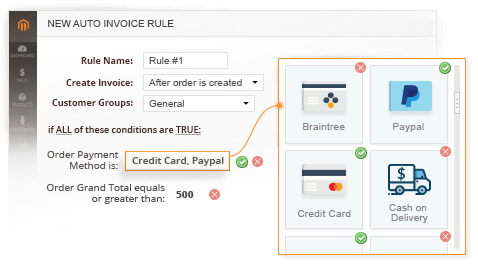
Flexible Rules
You can configure specific rules to specify when and how invoices and shipments are created automatically. These rules allow you to set conditions based on payment methods, order status, or other criteria, ensuring that your workflow aligns with your business needs.
Support for Partial Invoices and Shipments
If your business deals with partial shipments or split orders, the extension allows you to create partial invoices and shipments. This ensures that order statuses are updated correctly for each portion of the order that is fulfilled.
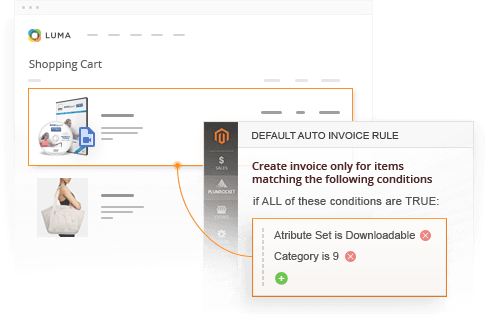
Automate the invoicing and shipping process to ensure order management efficiency and timely status updates.